java上传文件到指定服务器上,深入解析Java文件上传到指定服务器,技术实现与性能优化
- 综合资讯
- 2024-11-25 01:38:56
- 0
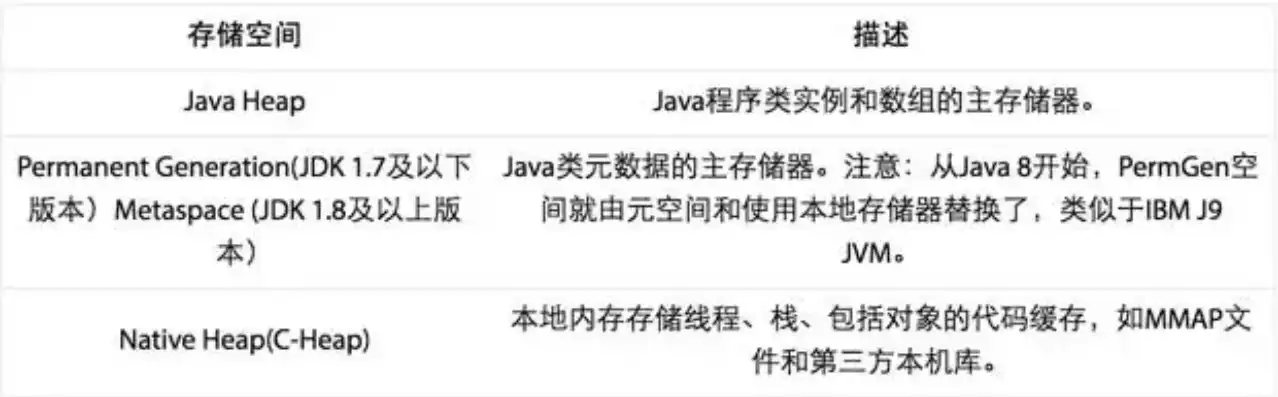
深入解析Java文件上传至指定服务器技术,包括实现细节和性能优化策略,涵盖文件选择、读取、传输至服务器及处理相关异常等环节,旨在提升文件上传效率和稳定性。...
深入解析Java文件上传至指定服务器技术,包括实现细节和性能优化策略,涵盖文件选择、读取、传输至服务器及处理相关异常等环节,旨在提升文件上传效率和稳定性。
随着互联网的快速发展,文件上传和下载已成为网络应用中不可或缺的功能,在Java开发中,上传文件到指定服务器是一项基本技能,本文将深入解析Java文件上传到指定服务器的过程,包括技术实现、性能优化以及注意事项。
技术实现
1、选择合适的上传方式
在Java中,上传文件到服务器主要有两种方式:表单上传和FTP上传。
(1)表单上传:通过HTML表单提交文件,使用HttpURLConnection或Spring MVC等技术实现。
(2)FTP上传:使用Java的java.net包中的FTPClient类实现。
以下以表单上传为例进行讲解。
2、编写上传代码
(1)HTML表单
<form action="upload" method="post" enctype="multipart/form-data"> <input type="file" name="file" /> <input type="submit" value="上传" /> </form>
(2)Java代码
import java.io.DataOutputStream; import java.io.File; import java.io.FileInputStream; import java.io.IOException; import java.net.HttpURLConnection; import java.net.URL; public class FileUpload { public static void main(String[] args) { String targetUrl = "http://example.com/upload"; File file = new File("path/to/your/file"); HttpURLConnection connection = null; DataOutputStream outputStream = null; FileInputStream inputStream = null; try { URL url = new URL(targetUrl); connection = (HttpURLConnection) url.openConnection(); connection.setRequestMethod("POST"); connection.setRequestProperty("Content-Type", "multipart/form-data"); connection.setDoOutput(true); outputStream = new DataOutputStream(connection.getOutputStream()); outputStream.writeBytes("filename=" + file.getName()); outputStream.writeBytes("&filedata="); inputStream = new FileInputStream(file); byte[] buffer = new byte[4096]; int bytesRead; while ((bytesRead = inputStream.read(buffer)) != -1) { outputStream.write(buffer, 0, bytesRead); } inputStream.close(); outputStream.flush(); outputStream.close(); if (connection.getResponseCode() == HttpURLConnection.HTTP_OK) { System.out.println("File uploaded successfully!"); } else { System.out.println("Failed to upload file."); } } catch (IOException e) { e.printStackTrace(); } finally { if (connection != null) { connection.disconnect(); } } } }
3、服务器端处理
服务器端需要根据上传的文件进行处理,以下以Spring Boot为例进行讲解。
import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.RestController; import org.springframework.web.multipart.MultipartFile; @RestController public class FileUploadController { @PostMapping("/upload") public String uploadFile(@RequestParam("file") MultipartFile file) { // 处理上传的文件 try { File serverFile = new File("path/to/save/" + file.getOriginalFilename()); file.transferTo(serverFile); return "File uploaded successfully!"; } catch (IOException e) { e.printStackTrace(); return "Failed to upload file."; } } }
性能优化
1、选择合适的上传方式
(1)表单上传:适用于小文件上传,但速度较慢。
(2)FTP上传:适用于大文件上传,速度快,但需要配置FTP服务器。
2、使用异步上传
对于大量文件上传,可以使用异步上传技术,提高用户体验。
3、优化服务器端处理
(1)使用缓存技术:对于频繁访问的文件,可以使用缓存技术,减少服务器压力。
(2)使用负载均衡:对于高并发上传,可以使用负载均衡技术,提高服务器处理能力。
注意事项
1、文件大小限制
在HTML表单中,可以使用<input type="file" accept="..." />
属性限制上传文件的类型。
2、安全问题
在文件上传过程中,需要注意文件类型、大小和内容的安全性问题,防止恶意攻击。
3、异常处理
在文件上传过程中,可能会遇到各种异常,需要做好异常处理,保证程序的健壮性。
本文深入解析了Java文件上传到指定服务器的技术实现、性能优化以及注意事项,在实际开发过程中,可以根据具体需求选择合适的上传方式,并进行性能优化,提高用户体验。
本文链接:https://www.zhitaoyun.cn/1052593.html
发表评论